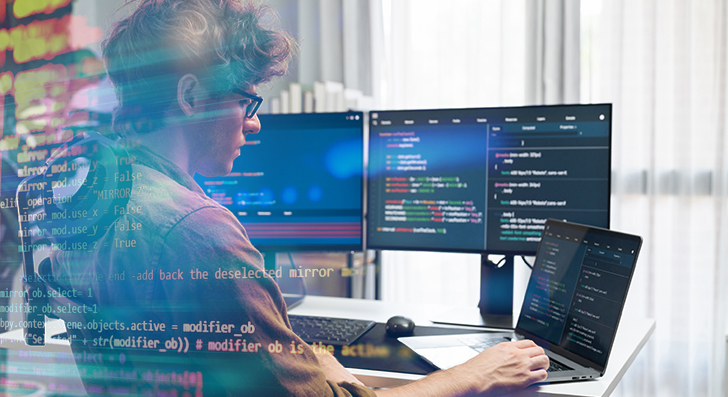
Scalability usually means your application can deal with growth—additional end users, a lot more data, and more targeted visitors—devoid of breaking. Being a developer, developing with scalability in your mind will save time and anxiety later. Below’s a clear and simple information that will help you get started by Gustavo Woltmann.
Style and design for Scalability from the beginning
Scalability is not a little something you bolt on later on—it ought to be element within your prepare from the start. A lot of programs are unsuccessful after they mature quickly because the initial design can’t take care of the additional load. Like a developer, you should Assume early about how your technique will behave stressed.
Begin by building your architecture to become versatile. Avoid monolithic codebases where by every thing is tightly linked. In its place, use modular style or microservices. These designs crack your application into smaller sized, impartial sections. Every single module or company can scale on its own without having impacting The complete method.
Also, think of your databases from day a single. Will it need to deal with 1,000,000 people or just a hundred? Select the ideal kind—relational or NoSQL—based upon how your details will increase. System for sharding, indexing, and backups early, Even when you don’t require them but.
A different vital point is to prevent hardcoding assumptions. Don’t compose code that only operates less than current circumstances. Think of what would transpire If the person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use design patterns that support scaling, like message queues or party-pushed devices. These enable your application take care of a lot more requests without the need of having overloaded.
Any time you Make with scalability in your mind, you're not just preparing for success—you might be cutting down long run problems. A very well-planned method is easier to take care of, adapt, and increase. It’s far better to prepare early than to rebuild afterwards.
Use the appropriate Database
Picking out the proper database is a key Component of constructing scalable programs. Not all databases are built a similar, and using the wrong one can gradual you down and even cause failures as your application grows.
Commence by understanding your facts. Is it really structured, like rows in the desk? If yes, a relational databases like PostgreSQL or MySQL is an effective in good shape. These are typically powerful with interactions, transactions, and consistency. In addition they assistance scaling procedures like browse replicas, indexing, and partitioning to deal with more targeted traffic and information.
In the event your info is a lot more flexible—like consumer activity logs, merchandise catalogs, or documents—look at a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at handling substantial volumes of unstructured or semi-structured information and might scale horizontally more very easily.
Also, take into consideration your go through and write designs. Will you be performing a great deal of reads with much less writes? Use caching and read replicas. Do you think you're managing a heavy compose load? Check into databases that can cope with high compose throughput, or maybe party-primarily based knowledge storage devices like Apache Kafka (for temporary information streams).
It’s also wise to Imagine ahead. You may not need to have State-of-the-art scaling features now, but choosing a database that supports them implies you gained’t need to have to modify afterwards.
Use indexing to speed up queries. Stay clear of unnecessary joins. Normalize or denormalize your information based on your accessibility designs. And constantly watch databases general performance when you grow.
In short, the appropriate databases will depend on your application’s framework, pace demands, And just how you assume it to increase. Just take time to choose properly—it’ll help you save loads of hassle afterwards.
Enhance Code and Queries
Rapidly code is vital to scalability. As your app grows, each small hold off provides up. Inadequately penned code or unoptimized queries can decelerate effectiveness and overload your technique. That’s why it’s crucial that you Construct effective logic from the start.
Get started by producing clear, easy code. Avoid repeating logic and take away just about anything unwanted. Don’t select the most complex Alternative if an easy a single works. Maintain your functions shorter, centered, and easy to test. Use profiling instruments to discover bottlenecks—places wherever your code will take too very long to run or takes advantage of excessive memory.
Subsequent, check out your database queries. These often sluggish things down in excess of the code itself. Ensure that Every question only asks for the data you really need. Keep away from SELECT *, which fetches almost everything, and rather choose precise fields. Use indexes to speed up lookups. And keep away from doing too many joins, In particular across huge tables.
For those who discover the exact same data getting requested over and over, use caching. Retail store the outcome quickly using equipment like Redis or Memcached this means you don’t must repeat high priced functions.
Also, batch your database operations if you can. In lieu of updating a row one after the other, update them in teams. This cuts down on overhead and makes your app far more successful.
Make sure to test with big datasets. Code and queries that operate high-quality with a hundred records may crash whenever they have to handle 1 million.
In brief, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when essential. These ways assistance your software stay smooth and responsive, whilst the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's to manage extra customers and even more website traffic. If all the things goes as a result of a person server, it'll rapidly become a bottleneck. That’s exactly where load balancing and caching come in. Both of these equipment aid maintain your app quick, stable, and scalable.
Load balancing spreads incoming traffic throughout many servers. As an alternative to one particular server undertaking every one of the perform, the load balancer routes customers to diverse servers depending on availability. This means no one server will get overloaded. If one particular server goes down, the load balancer can deliver traffic to the Many others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing info temporarily so it can be reused immediately. When end users request a similar data once more—like an item website page or even a profile—you don’t really need to fetch it through the database when. It is possible to serve it through the cache.
There are 2 typical different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for rapidly access.
two. Client-aspect caching (like browser caching or CDN caching) stores static documents near the consumer.
Caching cuts down databases load, improves pace, and makes your app extra effective.
Use caching for things which don’t alter typically. And usually ensure that your cache is updated when knowledge does change.
In a nutshell, load balancing and caching are simple but effective resources. Jointly, they assist your app take care of extra customers, keep speedy, and Recuperate from challenges. If you propose to develop, you require both.
Use Cloud and Container Resources
To develop scalable purposes, you require tools that let your app increase conveniently. That’s exactly where cloud platforms and containers are available in. They provide you overall flexibility, cut down set up time, and make scaling much smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you will need them. You don’t really need to obtain components or guess upcoming potential. When targeted visitors increases, you can include much more sources with only a few clicks or immediately making use of automobile-scaling. When site visitors drops, you'll be able to scale down to save money.
These platforms also offer you companies like managed databases, storage, get more info load balancing, and protection equipment. It is possible to target constructing your app rather than managing infrastructure.
Containers are another key Software. A container offers your app and every thing it must operate—code, libraries, configurations—into one particular device. This causes it to be simple to maneuver your application among environments, from your notebook to your cloud, with no surprises. Docker is the most popular tool for this.
Once your app uses various containers, equipment like Kubernetes assist you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single element of your application crashes, it restarts it instantly.
Containers also make it very easy to separate aspects of your app into services. You may update or scale elements independently, which happens to be great for performance and dependability.
In short, working with cloud and container resources usually means you'll be able to scale speedy, deploy conveniently, and Recuperate promptly when issues materialize. If you'd like your application to develop devoid of limits, start off using these resources early. They help you save time, decrease chance, and help you remain centered on building, not fixing.
Watch Everything
When you don’t keep track of your application, you gained’t know when matters go Incorrect. Monitoring can help the thing is how your app is executing, place difficulties early, and make better choices as your app grows. It’s a essential Element of building scalable techniques.
Start off by monitoring essential metrics like CPU usage, memory, disk Room, and reaction time. These inform you how your servers and products and services are doing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you acquire and visualize this facts.
Don’t just watch your servers—observe your application too. Keep an eye on how long it takes for customers to load pages, how often mistakes occur, and exactly where they take place. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will help you see what’s taking place within your code.
Put in place alerts for critical troubles. By way of example, If the reaction time goes previously mentioned a limit or even a services goes down, you need to get notified instantly. This helps you fix challenges speedy, generally ahead of end users even recognize.
Monitoring is usually handy any time you make alterations. Should you deploy a fresh function and find out a spike in glitches or slowdowns, it is possible to roll it back right before it will cause actual damage.
As your application grows, targeted traffic and information maximize. With no monitoring, you’ll pass up signs of trouble until eventually it’s also late. But with the right instruments in place, you continue to be in control.
To put it briefly, monitoring helps you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about comprehension your system and making certain it works properly, even under pressure.
Ultimate Thoughts
Scalability isn’t just for significant organizations. Even compact apps will need a strong Basis. By designing meticulously, optimizing sensibly, and using the suitable resources, you may Develop apps that mature smoothly without the need of breaking under pressure. Start out small, Feel significant, and Develop sensible.